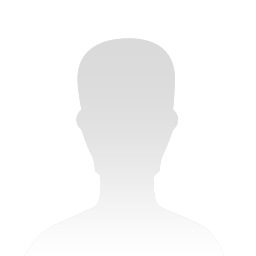
Master the Linux bash command line with these 11 shortcuts
Posted by emilio.moretti in Emilio Moretti's Blog on May 31, 2011 12:44:25 PMIfyou've ever typed a command at the Linux shell prompt, you've probably already used bash—after all,it's the default command shell on most modern GNU/Linux distributions. The bash shell is the primary interface to the Linux operating system—it accepts, interprets and executes your commands,and provides you with the building blocks for shell scripting and automated task execution.
bash's unassuming exterior hides some very powerful tools and shortcuts. If you're a heavy user of the command line, these can save you a fair bit of typing. This document outlines ten of the most useful tools:
1. Easily recall previous commands
bash keeps trackof the commands you execute in a history buffer, and allows you to recall previous commands by cycling through them with the Up and Down cursor keys.For even faster recall, "speed search" previously-executed commands by typing the first few letters of the command followed by the key combination Ctrl-R; bash will then scan the command history for matching commands and display them on the console.Type Ctrl-R repeatedly to cycle through the entire list of matching commands.
2. Use command aliases
Ifyou always run a command with the same set of options, you can have bash create an alias for it. This alias will incorporate the required options, so that you don't need to remember them or manually type them every time. For example, ifyou always run ls with the -l option to obtain a detailed directory listing, you can use this command:
bash> alias ls='ls -l'
To create an alias that automatically includes the -l option. Once this alias has been created, typing ls at the bash prompt will invoke the alias and produce the ls -l output.
You can obtain a list of available aliases by invoking alias without any arguments, and you can delete an alias with unalias.
3. Use filename auto-completion
bash supports filename auto-completion at the command prompt. To use this feature, type the first few letters of the file name, followed by Tab. bash will scan the current directory, as well as all other directories in the search path, for matches to that name. If a single match is found, bash will automatically complete the filename for you. If multiple matches are found, you will be prompted to choose one.
4. Use key shortcuts to efficiently edit the command line
bash supports a number of keyboard shortcuts for command-line navigation and editing. The Ctrl-A key shortcut moves the cursor to the beginning of the command line, while the Ctrl-E shortcut moves the cursor to the end of the command line. The Ctrl-W shortcut deletes the word immediately before the cursor, while the Ctrl-K shortcut deletes everything immediately after the cursor. You can undo a deletion with Ctrl-Y.
5. Get automatic notification of new mail
You can configure bash to automatically notify you of new mail, by setting the $MAILPATH variable to point to your local mail spool. For example, the command:
bash> MAILPATH='/var/spool/mail/john'
bash> export MAILPATH
Causes bash to print a notification on john's console every time a new message is appended to john's mail spool.
6. Run tasks in the background
bash lets you run one or more tasks in the background, and selectively suspend or resume any of the current tasks (or "jobs"). To run a task in the background, add an ampersand (&) to the end of its command line. Here's an example:
bash> tail -f /var/log/messages &
[1] 614
Each task back-grounded in this manner in assigned a job ID, which is printed to the console. A task can be brought back to the foreground with the command fg jobnumber, where jobnumber is the job IDof the task you wish to bring to the foreground. Here's an example:
bash> fg 1
Alist of active jobs can be obtained at any time by typing jobs at the bash prompt.
7. Quickly jump to frequently-used directories
You probably already know that the $PATH variable lists bash's "search path" - the directories it will search when it can't find the requested file in the current directory. However, bash also supports the $CDPATH variable,which lists the directories the cd command will look in when attempting to change directories. To use this feature, assign a directory list to the $CDPATH variable, as shown in the example below:
bash> CDPATH='.:~:/usr/local/apache/htdocs:/disk1/backups'
bash> export CDPATH
Now,whenever you use the cd command, bash will check all the directories in the $CDPATH list for matches to the directory name.
8. Perform calculations
bash can perform simple arithmetic operations at the command prompt. To use this feature, simply type in the arithmetic expression you wish to evaluate at the prompt within double parentheses, as illustrated below. bash will attempt to perform the calculation and return the answer.
bash> echo $((16/2))
8
9. Customize the shell prompt
You can customize the bash shell prompt to display—among other things—the current username and host name, the current time, the load average and/or the current working directory. To do this, alter the $PS1 variable, as below:
bash> PS1='\u@\h:\w \@> '
bash> export PS1
root@medusa:/tmp 03:01 PM>
This will display the name of the currently logged-in user, the host name, thecurrent working directory and the current time at theshell prompt. You can obtain a list of symbols understood by bash from its manual page.
10. Get context-specific help
bash comes with help for all built-in commands. To see a list of all built-in commands, type help. To obtain help on a specific command,type help command, where command is the command you need help on. Here's an example:
bash> help alias
...some help text...
Obviously,you can obtain detailed help on the bash shell by typing man bash at your command prompt at any time.
11. Execute several commands in a single line
Use the double-ampersand operator in bash to provide conditional execution: $ cd mytmp && rm * Two commands separated by the double ampersands tells bash to run the first command and then to run the second command only if the first command succeeds (i.e., its exit status is 0). This is very much like using an if statement to check the exit status of the first command in order to protect the running of the second command: cd mytmp The double ampersand syntax is meant to be reminiscent of the logical and operator in C Language. If you know your logic (and your C) then you’ll recall that if you are evaluating the logical expression A AND B, then the entire expression can only be true if both (sub)expression A and (sub)expression B evaluate to true. If either one is false, the whole expression is false. C Language makes use of this fact, and when you code an expression likeif (A && B) { ... }, it will evaluate expressionAfirst. If it is false, it won’t even bother to evaluateBsince the overall outcome (false) has already been determined (by A being false). So what does this have to do with bash? Well, if the exit status of the first command (the one to the left of the &&) is non-zero (i.e., failed) then it won’t bother to evaluate the second expression—i.e., it won’t run the other command at all. If you want to be thorough about your error checking, but don’t want if statements all over the place, you can have bash exit any time it encounters a failure (i.e., a non-zero exit status) from every command in your script (except in while loops and if statements where it is already capturing and using the exit status) by setting the -e flag. set -e Setting the -e flag will cause the shell to exit when a command fails. If the cd fails, the script will exit and never even try to execute the rm * command. We don’t recommend doing this on an interactive shell, because when the shell exits it will make your shell window go away. Sources: http://www.devshed.com/c/a/BrainDump/Better-Command-Execution-with-bash/
if (( $? )); then rm * ; fi
cd mytmp
rm *
Comments